In Android, you can use
See following code snippets :
P.S This project is developed in Eclipse 3.7, and tested with Samsung Galaxy S2 (Android 2.3.3).
It will prompts your existing Email client to select.
In this case, i selected Gmail, and all previous filled in detail will be populated to Gmail client automatically.
Intent.ACTION_SEND
to call an existing email client to send an Email.See following code snippets :
Intent email = new Intent(Intent.ACTION_SEND); email.putExtra(Intent.EXTRA_EMAIL, new String[]{"youremail@yahoo.com"}); email.putExtra(Intent.EXTRA_SUBJECT, "subject"); email.putExtra(Intent.EXTRA_TEXT, "message"); email.setType("message/rfc822"); startActivity(Intent.createChooser(email, "Choose an Email client :"));
Run & test on real device only.
If you run this on emulator, you will hit error message : “No application can perform this action“. This code only work on real device.
If you run this on emulator, you will hit error message : “No application can perform this action“. This code only work on real device.
1. Android Layout
File : res/layout/main.xml<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/linearLayout1" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:id="@+id/textViewPhoneNo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="To : " android:textAppearance="?android:attr/textAppearanceLarge" /> <EditText android:id="@+id/editTextTo" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="textEmailAddress" > <requestFocus /> </EditText> <TextView android:id="@+id/textViewSubject" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Subject : " android:textAppearance="?android:attr/textAppearanceLarge" /> <EditText android:id="@+id/editTextSubject" android:layout_width="fill_parent" android:layout_height="wrap_content" > </EditText> <TextView android:id="@+id/textViewMessage" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Message : " android:textAppearance="?android:attr/textAppearanceLarge" /> <EditText android:id="@+id/editTextMessage" android:layout_width="fill_parent" android:layout_height="wrap_content" android:gravity="top" android:inputType="textMultiLine" android:lines="5" /> <Button android:id="@+id/buttonSend" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Send" /> </LinearLayout>
2. Activity
Full activity class to send an Email. Read theonClick()
method, it should be self-explanatory.package com.mkyong.android; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; public class SendEmailActivity extends Activity { Button buttonSend; EditText textTo; EditText textSubject; EditText textMessage; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); buttonSend = (Button) findViewById(R.id.buttonSend); textTo = (EditText) findViewById(R.id.editTextTo); textSubject = (EditText) findViewById(R.id.editTextSubject); textMessage = (EditText) findViewById(R.id.editTextMessage); buttonSend.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { String to = textTo.getText().toString(); String subject = textSubject.getText().toString(); String message = textMessage.getText().toString(); Intent email = new Intent(Intent.ACTION_SEND); email.putExtra(Intent.EXTRA_EMAIL, new String[]{ to}); //email.putExtra(Intent.EXTRA_CC, new String[]{ to}); //email.putExtra(Intent.EXTRA_BCC, new String[]{to}); email.putExtra(Intent.EXTRA_SUBJECT, subject); email.putExtra(Intent.EXTRA_TEXT, message); //need this to prompts email client only email.setType("message/rfc822"); startActivity(Intent.createChooser(email, "Choose an Email client :")); } }); } }
3. Demo
See default scree, fill in the detail, and click on the “send” button.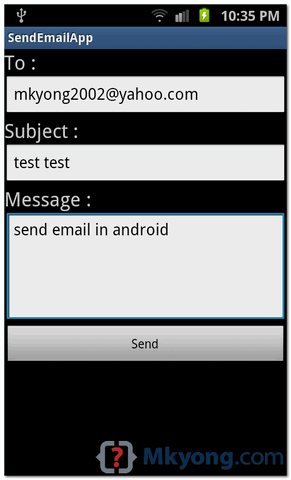
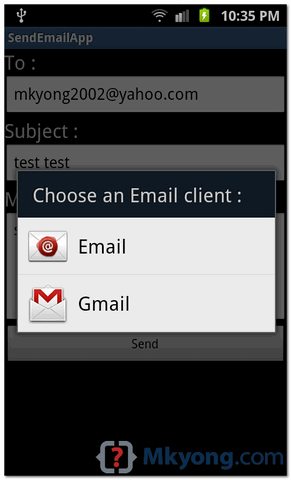
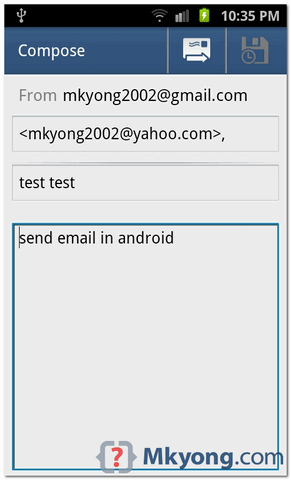
Note
Android does not provide API to send Email directly, you have to call the existing Email client to send Email.
Android does not provide API to send Email directly, you have to call the existing Email client to send Email.
Download Source Code
Download it – Android-Send-Email-Example.zip (16 KB)
jianbin1205
ReplyDeletecheap uggs
north face outlet online
coach outlet online
uggs outlet
snapback hats
true religion outlet,true religion jeans,true religion kids,true religion jeans sale,true religion jeans for men,true religion jacket,true religion sale
cheap oakley sunglasses
moncler coats
ugg boots on sale
cyber monday
kobe bryants shoes 2015
polo ralph lauren uk
moncler outlet
lululemon outlet
prada outlet
chanel handbags
ugg uk outlet
toms outlet
canada goose outlet
the north face jackets
new england patriots
chicago blackhawks
michael kors outlet sale
new york giants
air force one shoes
nike air max 90
ugg boots
air jordan 11 free shipping
michael kors handbags
nike trainers uk
air jordan shoes
kansas city chiefs
chicago bulls
For most business owners, making the decision to implement an IVR or voice response software is easy. Here are some tips for choosing IVRs and providers that will benefit you the most.Hosted IVR
ReplyDeletechenenen0506
ReplyDeletetory burch boots
jordan retro 11
nike trainers uk
air force 1
cheap toms
louis vuitton outlet
kate spade outlet
louis vuitton outlet stores
true religion jeans
celine handbags
nike store
air max 90
jordan retro 8
coach outlet
louis vuitton purses
cheap jordans
hollister uk
tory burch outlet online
pandora outlet
fitflops shoes
michael kors outlet
jordan 11
discount jordans
louis vuitton outlet
hollister
christian louboutin shoes
kobe 10
gucci handbags
christian louboutin sale
fit flops
hogan outlet
ReplyDeleteyeezys
curry 6
christian louboutin shoes
kd shoes
jordan retro
polo ralph lauren
air max 97
canada goose jacket
jordans