In JSF, “h:selectOneRadio” tag is used to render a set of HTML input element of type “radio“, and format it with HTML table and label tag.
result.xhtml…
When “submit” button is clicked, link to “result.xhtml” and display the submitted radio button values.
The “favColor2″ radio button, “Red” option is selected by default.
//JSF... <h:selectOneRadio value="#{user.favColor1}"> <f:selectItem itemValue="Red" itemLabel="Color1 - Red" /> <f:selectItem itemValue="Green" itemLabel="Color1 - Green" /> <f:selectItem itemValue="Blue" itemLabel="Color1 - Blue" /> </h:selectOneRadio> //HTML output... <table> <tr> <td> <input type="radio" name="j_idt6:j_idt8" id="j_idt6:j_idt8:0" value="Red" /> <label for="j_idt6:j_idt8:0"> Color1 - Red</label></td> <td> <input type="radio" name="j_idt6:j_idt8" id="j_idt6:j_idt8:1" value="Green" /> <label for="j_idt6:j_idt8:1"> Color1 - Green</label></td> <td> <input type="radio" name="j_idt6:j_idt8" id="j_idt6:j_idt8:2" value="Blue" /> <label for="j_idt6:j_idt8:2"> Color1 - Blue</label> </td> </tr> </table>
JSF 2.0 “h:selectOneRadio” example
A JSF 2.0 example to show the use of “h:selectOneRadio” tag to render radio buttons, and populate the data in 3 different ways :- Hardcoded values in “f:selectItem” tag.
- Generate values with a Map and put it into “f:selectItems” tag.
- Generate values with an Object array and put it into “f:selectItems” tag, then represent the value with a “var” attribute.
1. Backing Bean
A backing bean to hold the submitted data.package com.mkyong; import java.util.LinkedHashMap; import java.util.Map; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; @ManagedBean(name="user") @SessionScoped public class UserBean{ public String favColor1; public String favColor2; public String favColor3; //getter and setter methods //Generated by Map private static Map<String,Object> color2Value; static{ color2Value = new LinkedHashMap<String,Object>(); color2Value.put("Color2 - Red", "Red"); //label, value color2Value.put("Color2 - Green", "Green"); color2Value.put("Color3 - Blue", "Blue"); } public Map<String,Object> getFavColor2Value() { return color2Value; } //Generated by Object array public static class Color{ public String colorLabel; public String colorValue; public Color(String colorLabel, String colorValue){ this.colorLabel = colorLabel; this.colorValue = colorValue; } public String getColorLabel(){ return colorLabel; } public String getColorValue(){ return colorValue; } } public Color[] color3List; public Color[] getFavColor3Value() { color3List = new Color[3]; color3List[0] = new Color("Color3 - Red", "Red"); color3List[1] = new Color("Color3 - Green", "Green"); color3List[2] = new Color("Color3 - Blue", "Blue"); return color3List; } }
2. JSF Page
A JSF page to demonstrate the use “h:selectOneRadio” tag.<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core" > <h:body> <h1>JSF 2 radio button example</h1> <h:form> 1. Hard-coded with "f:selectItem" : <h:selectOneRadio value="#{user.favColor1}"> <f:selectItem itemValue="Red" itemLabel="Color1 - Red" /> <f:selectItem itemValue="Green" itemLabel="Color1 - Green" /> <f:selectItem itemValue="Blue" itemLabel="Color1 - Blue" /> </h:selectOneRadio> <br /> 2. Generated by Map : <h:selectOneRadio value="#{user.favColor2}"> <f:selectItems value="#{user.favColor2Value}" /> </h:selectOneRadio> <br /> 3. Generated by Object array and iterate with var : <h:selectOneRadio value="#{user.favColor3}"> <f:selectItems value="#{user.favColor3Value}" var="c" itemLabel="#{c.colorLabel}" itemValue="#{c.colorValue}" /> </h:selectOneRadio> <br /> <h:commandButton value="Submit" action="result" /> <h:commandButton value="Reset" type="reset" /> </h:form> </h:body> </html>
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" > <h:body> <h1>JSF 2 radio button example</h1> <h2>result.xhtml</h2> <ol> <li>user.favColor1 : #{user.favColor1}</li> <li>user.favColor2 : #{user.favColor2}</li> <li>user.favColor3 : #{user.favColor3}</li> </ol> </h:body> </html>
3. Demo
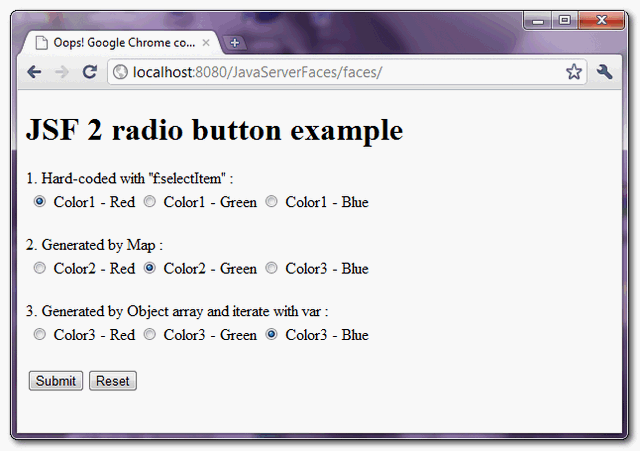
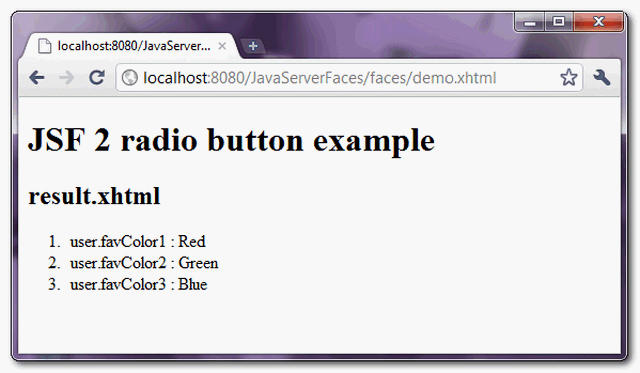
How to select radio button value by default?
In JSF, the radio button values of “f:selectItems” tag is selected if it matched to the “value” of “h:selectOneRadio” tag. In above example, if you set favColor2 to “Red” :@ManagedBean(name="user") @SessionScoped public class UserBean{ public String[] favColor = "Red"; //...
Download Source Code
Download It – JSF-2-RadioButtons-Example.zip (10KB)
JSF2 radio buttons example is awesome and is to get more knowledge about the radio buttons in case of coding.It is nice blog.
ReplyDeletejava training in chennai
Really it was an awesome article...very interesting to read..You have provided an nice article....Thanks for sharing.
ReplyDeleteBest BCA Colleges in Noida
Faisalabad Fabric Store the name where you can buy Ladies Velvet Designer Suits either in Casual wear or in Party Wear. Faisalabadfabricstore.com has propelled its designer velvet designs replica collection in this season. Having the latest designs of different brands like Khaadi, Sana Safinaz, Asim Jofa, Maria B, Ethnic, Tena Durrani and some more you can find a lot of designs and styles in a single place.
ReplyDeletevelvet kurta for ladies , velvet punjabi suits designs , velvet punjabi suit
JSF2 radio buttons example is awesome and is to get more knowledge about the radio buttons in case of coding.It is nice blog.
ReplyDeleteshawls for dresses , shawls for women , shawls for sale , black shawl , white shawl , shawl , silver shawl , gold shawl , navy shawl , woolen shawl
vans outlet
ReplyDeleteair jordan
goyard
lebron 15 shoes
canada goose
curry 6 shoes
air max 270
balenciaga speed
supreme t shirt
fila shoes
JSF2 radio buttons example is awesome and is to get more knowledge about the radio buttons in case of coding.It is nice blog. antique coin necklace , best handmade shoes , anklet jewelry , embroidery purses online , black belt embroidery taekwondo , mens jean belts , jean boots with belt , charm bracelets
ReplyDelete