JSF dataTable does not contains any method to display the currently selected row numbers. However, you can hack it with javax.faces.model.DataModel class, which has a getRowIndex() method to return the currently selected row number.
JSF + DataModel
Here’s a JSF 2.0 example to show you how to use DataModel to return the currently selected row number.1. Managed Bean
A managed bean named “person”, and show the use of DataModel to hold a list of the person object.package com.mkyong; import java.io.Serializable; import javax.faces.bean.ManagedBean; import javax.faces.bean.SessionScoped; import javax.faces.model.ArrayDataModel; import javax.faces.model.DataModel; @ManagedBean(name="person") @SessionScoped public class PersonBean implements Serializable{ private static final long serialVersionUID = 1L; private static final Person[] personList = new Person[]{ new Person("Person", "A", 10), new Person("Person", "B", 20), new Person("Person", "C", 30), new Person("Person", "D", 40), new Person("Person", "E", 50) }; /* To get the row numbers, use dataModel instead public Person[] getPersonList() { return personList; } */ private DataModel<Person> person = new ArrayDataModel<Person>(personList); public DataModel<Person> getPersonList() { return person; } public static class Person{ String firstName; String lastName; int age; public Person(String firstName, String lastName, int age) { super(); this.firstName = firstName; this.lastName = lastName; this.age = age; } //getter and setter methods } }
2. JSF Page
JSP page to show the use of DataModel “rowIndex” to return 0-indexed of the currently selected row number.<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core" xmlns:ui="http://java.sun.com/jsf/facelets" > <h:head> <h:outputStylesheet library="css" name="table-style.css" /> </h:head> <h:body> <h1>Display dataTable row numbers in JSF</h1> <h:dataTable value="#{person.personList}" var="p" styleClass="person-table" headerClass="person-table-header" rowClasses="person-table-odd-row,person-table-even-row" > <h:column> <!-- display currently selected row number --> <f:facet name="header">No</f:facet> #{person.personList.rowIndex + 1} </h:column> <h:column> <f:facet name="header">First Name</f:facet> #{p.firstName} </h:column> <h:column> <f:facet name="header">Last Name</f:facet> #{p.lastName} </h:column> <h:column> <f:facet name="header">Age</f:facet> #{p.age} </h:column> </h:dataTable> </h:body> </html>
3. Demo
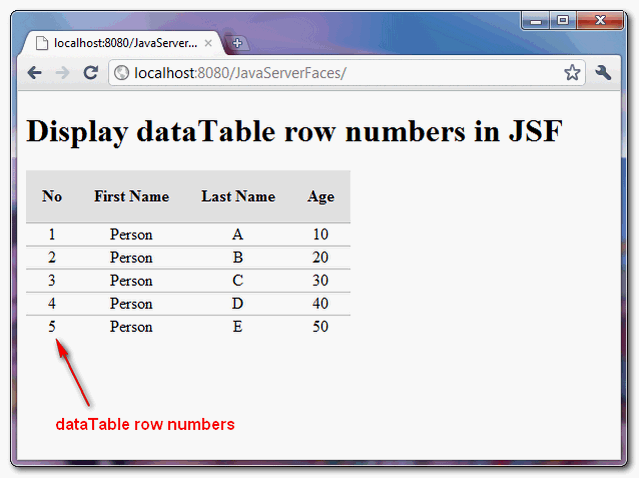
Download Source Code
Download It – JSF-2-DataTable-RowNumbers-Example.zip (10KB)
Burberry Outlet
ReplyDeleteOakley Eyeglasses Michael Kors Outlet Coach Factory Outlet Coach Outlet Online Coach Purses Kate Spade Outlet Toms Shoes North Face Outlet Coach Outlet Gucci Belt North Face Jackets Oakley Sunglasses Toms Outlet North Face Outlet Nike Outlet Nike Hoodies Tory Burch Flats Marc Jacobs Handbags Jimmy Choo Shoes Jimmy Choos
Burberry Belt Tory Burch Boots Louis Vuitton Belt Ferragamo Belt Marc Jacobs Handbags Lululemon Outlet Christian Louboutin Shoes True Religion Outlet Tommy Hilfiger Outlet
Michael Kors Outlet Coach Outlet Red Bottoms Kevin Durant Shoes New Balance Outlet Adidas Outlet Coach Outlet Online Stephen Curry Jersey
Skechers Go Walk Adidas Yeezy Boost Adidas Yeezy Adidas NMD Coach Outlet North Face Outlet Ralph Lauren Outlet Puma SneakersPolo Outlet
ReplyDeleteUnder Armour Outlet Under Armour Hoodies Herve Leger MCM Belt Nike Air Max Louboutin Heels Jordan Retro 11 Converse Outlet Nike Roshe Run UGGS Outlet North Face Outlet
Adidas Originals Ray Ban Lebron James Shoes Sac Longchamp Air Max Pas Cher Chaussures Louboutin Keds Shoes Asics Shoes Coach Outlet Salomon Shoes True Religion Outlet
New Balance Outlet Skechers Outlet Nike Outlet Adidas Outlet Red Bottom Shoes New Jordans Air Max 90 Coach Factory Outlet North Face Jackets North Face Outlet
Really it was an awesome article...very interesting to read..You have provided an nice article....Thanks for sharing.
ReplyDeleteBest BCA Colleges in Noida
longchamp handbags
ReplyDeletegolden goose sneakers
golden goose sneakers
supreme clothing
supreme clothing
kyrie 7 shoes
kobe
stone island sale
nike x off white
kenzo