In Android, you can use “android.widget.DatePicker” class to render a date picker component to select day, month and year in a pre-defined user interface.
In this tutorial, we show you how to render date picker component in current page via android.widget.DatePicker, and also in dialog box via android.app.DatePickerDialog. In addition, we also show you how to set a date in date picker component.
P.S This project is developed in Eclipse 3.7, and tested with Android 2.3.3.
File : res/layout/main.xml
P.S The “
File : MyAndroidAppActivity.java
P.S The “DatePickerDialog” example above, is referenced from Google Android date picker example, with some minor change.
1. Result, “date picker” and “textview” are set to current date.
2. Click on the “Change Date” button, it will prompt a date picker component in a dialog box via
3. Both “date picker” and “textview” are updated with selected date.
In this tutorial, we show you how to render date picker component in current page via android.widget.DatePicker, and also in dialog box via android.app.DatePickerDialog. In addition, we also show you how to set a date in date picker component.
P.S This project is developed in Eclipse 3.7, and tested with Android 2.3.3.
1. DatePicker
Open “res/layout/main.xml” file, add date picker, label and button for demonstration.File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <Button android:id="@+id/btnChangeDate" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Change Date" /> <TextView android:id="@+id/lblDate" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Current Date (M-D-YYYY): " android:textAppearance="?android:attr/textAppearanceLarge" /> <TextView android:id="@+id/tvDate" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="" android:textAppearance="?android:attr/textAppearanceLarge" /> <DatePicker android:id="@+id/dpResult" android:layout_width="wrap_content" android:layout_height="wrap_content" /> </LinearLayout>
DatePickerDialog
” is declare in code, not XML.2. Code Code
Read the code’s comment, it should be self-explanatory.File : MyAndroidAppActivity.java
package com.mkyong.android; import java.util.Calendar; import android.app.Activity; import android.app.DatePickerDialog; import android.app.Dialog; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.DatePicker; import android.widget.TextView; public class MyAndroidAppActivity extends Activity { private TextView tvDisplayDate; private DatePicker dpResult; private Button btnChangeDate; private int year; private int month; private int day; static final int DATE_DIALOG_ID = 999; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); setCurrentDateOnView(); addListenerOnButton(); } // display current date public void setCurrentDateOnView() { tvDisplayDate = (TextView) findViewById(R.id.tvDate); dpResult = (DatePicker) findViewById(R.id.dpResult); final Calendar c = Calendar.getInstance(); year = c.get(Calendar.YEAR); month = c.get(Calendar.MONTH); day = c.get(Calendar.DAY_OF_MONTH); // set current date into textview tvDisplayDate.setText(new StringBuilder() // Month is 0 based, just add 1 .append(month + 1).append("-").append(day).append("-") .append(year).append(" ")); // set current date into datepicker dpResult.init(year, month, day, null); } public void addListenerOnButton() { btnChangeDate = (Button) findViewById(R.id.btnChangeDate); btnChangeDate.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { showDialog(DATE_DIALOG_ID); } }); } @Override protected Dialog onCreateDialog(int id) { switch (id) { case DATE_DIALOG_ID: // set date picker as current date return new DatePickerDialog(this, datePickerListener, year, month,day); } return null; } private DatePickerDialog.OnDateSetListener datePickerListener = new DatePickerDialog.OnDateSetListener() { // when dialog box is closed, below method will be called. public void onDateSet(DatePicker view, int selectedYear, int selectedMonth, int selectedDay) { year = selectedYear; month = selectedMonth; day = selectedDay; // set selected date into textview tvDisplayDate.setText(new StringBuilder().append(month + 1) .append("-").append(day).append("-").append(year) .append(" ")); // set selected date into datepicker also dpResult.init(year, month, day, null); } }; }
3. Demo
Run the application.1. Result, “date picker” and “textview” are set to current date.
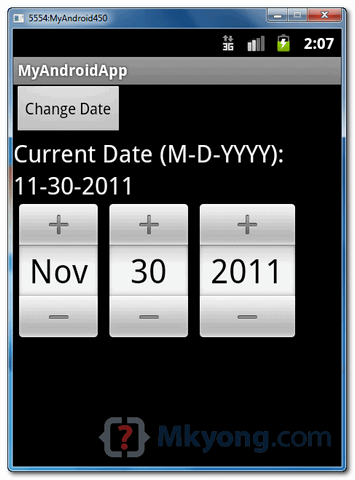
DatePickerDialog
. 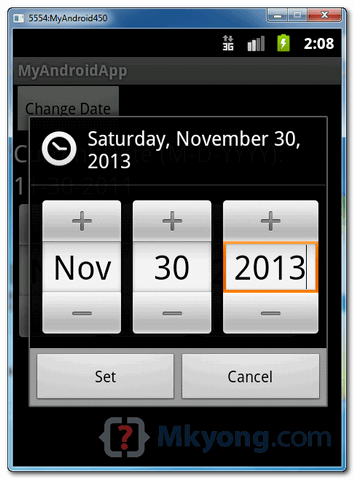
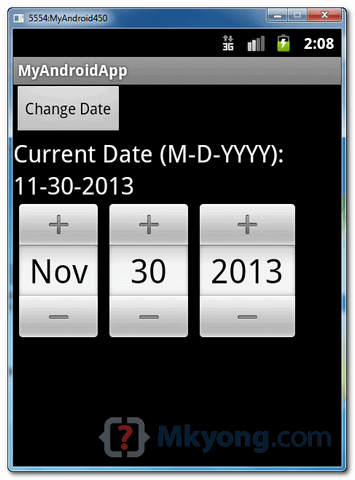
Download Source Code
Download it – Android-DatePicker-Example.zip (16 KB)
Perfect thanks... This http://www.compiletimeerror.com/2013/07/android-date-picker-example-android.html looks a bit different, may help.. Have a look...
ReplyDeletethank you Meghana ..!!
DeleteYou should write a source in your artcles... This is mkyong 's post....
ReplyDeleteYou just reposted this verbatim from another website, and it isn't even accurate anymore. The methods used are depreciated.
ReplyDeleteThis comment has been removed by the author.
ReplyDeletecheck for important android interview questions @ http://skillgun.com
ReplyDeleteAndroid Tutorial Basic to Advance: Click Here
ReplyDeleteAndroid User Interface Tutorial: Click Here
All are saying the same thing repeatedly, but in your blog I had a chance to get some useful and unique information, I love your writing style very much, I would like to suggest your blog in my dude circle, so keep on updates.
ReplyDeletePeridot Systems Chennai Contact Number
Nice looking sites and great work. Pretty nice information. it has a better understanding. thanks for spending time on it.
ReplyDeleteBest Industrial Training in Noida
Best Industrial Training in Noida
Everythings Works Perfect!
ReplyDeleteBest BCA Colleges in Noida
off white jordan 1
ReplyDeletekobe 9
yeezy boost
coach bags
coach factory outlet
golden goose outlet
curry 4 shoes
nike air max
bape hoodie
yeezy boost
this contentimportant site More about the authorMore about the author useful referenceimportant source
ReplyDeletearticle source web investigate this site try this website Home Page Clicking Here
ReplyDeleteimp source Bottega Veneta Dolabuy their explanation Louis Vuitton fake Bags i loved this replica bags
ReplyDeletereplica bags south africa Discover More Here g0a54z4h15 replica bags los angeles 9a replica bags gucci fake j1t67r5d31 replica bags wholesale mumbai Full Article z0a01f1r96 replica louis vuitton joy replica bags review
ReplyDeleten5i98g4v29 c3h73c1r30 h6l00e4v17 d9h33g5k75 c1o13h5o93 v2b98d6r97
ReplyDeleteİstanbul
ReplyDeleteSivas
Kırıkkale
Zonguldak
Iğdır
LH2E
van
ReplyDeletekastamonu
elazığ
tokat
sakarya
65C
C634A
ReplyDeleteErzurum Evden Eve Nakliyat
Isparta Evden Eve Nakliyat
Karaman Evden Eve Nakliyat
Ankara Evden Eve Nakliyat
Malatya Evden Eve Nakliyat
FCC32
ReplyDeleteHotbit Güvenilir mi
Siirt Lojistik
Çerkezköy Yol Yardım
Tokat Parça Eşya Taşıma
Van Şehirler Arası Nakliyat
Osmaniye Evden Eve Nakliyat
Bitcoin Nasıl Alınır
Antalya Şehir İçi Nakliyat
Rize Evden Eve Nakliyat
92480
ReplyDeleteSivas Şehir İçi Nakliyat
Çorum Şehirler Arası Nakliyat
Niğde Evden Eve Nakliyat
Bilecik Lojistik
Ağrı Parça Eşya Taşıma
Keçiören Fayans Ustası
Caw Coin Hangi Borsada
Hotbit Güvenilir mi
Adana Lojistik
811AE
ReplyDeletebinance %20
3D9EF
ReplyDeleteGörüntülü Sohbet Parasız
Tiktok İzlenme Hilesi
Binance Borsası Güvenilir mi
Bitcoin Nasıl Oynanır
Kripto Para Üretme
Binance Hesap Açma
Kaspa Coin Hangi Borsada
Linkedin Takipçi Satın Al
Facebook Takipçi Satın Al
C6ACC
ReplyDeleteuniswap
dextools
dcent
defilama
pancakeswap
ellipal
chainlist
avax
looksrare
31D79AC6FB
ReplyDeletetürk takipci
9C71917886
ReplyDeleteçekilişle takipçi
Razer Gold Promosyon Kodu
101 Okey Vip Hediye Kodu
MMORPG Oyunlar
Pokemon GO Promosyon Kodu
Pasha Fencer Hediye Kodu
İdle Office Tycoon Hediye Kodu
Pubg New State Promosyon Kodu
Erasmus Proje