In Android, you can use “android.widget.EditText“, with
In this tutorial, we show you how to use XML to create a password field, label field and a normal button. When you click on the button, the password value will be displayed as a floating message (toast message).
P.S This project is developed in Eclipse 3.7, and tested with Android 2.3.3.
File : res/values/strings.xml
File : res/layout/main.xml
File : MyAndroidAppActivity.java
1. Result, password field is displayed.
2. Type password “mkyong123″ and click on the submit button.
inputType="textPassword"
to render a password component.In this tutorial, we show you how to use XML to create a password field, label field and a normal button. When you click on the button, the password value will be displayed as a floating message (toast message).
P.S This project is developed in Eclipse 3.7, and tested with Android 2.3.3.
1. Custom String
Open “res/values/strings.xml” file, add some custom string for demonstration.File : res/values/strings.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="app_name">MyAndroidApp</string> <string name="lblPassword">Enter Your Password :</string> <string name="btn_submit">Submit</string> </resources>
2. Password
Open “res/layout/main.xml” file, add a password component,EditText
+ inputType="textPassword"
.File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:id="@+id/lblPassword" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/lblPassword" android:textAppearance="?android:attr/textAppearanceLarge" /> <EditText android:id="@+id/txtPassword" android:layout_width="match_parent" android:layout_height="wrap_content" android:inputType="textPassword" > <requestFocus /> </EditText> <Button android:id="@+id/btnSubmit" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="@string/btn_submit" /> </LinearLayout>
3. Code Code
Inside activity “onCreate()
” method, attach a click listener on button, to display the password value.File : MyAndroidAppActivity.java
package com.mkyong.android; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class MyAndroidAppActivity extends Activity { private EditText password; private Button btnSubmit; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); addListenerOnButton(); } public void addListenerOnButton() { password = (EditText) findViewById(R.id.txtPassword); btnSubmit = (Button) findViewById(R.id.btnSubmit); btnSubmit.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { Toast.makeText(MyAndroidAppActivity.this, password.getText(), Toast.LENGTH_SHORT).show(); } }); } }
4. Demo
Run the application.1. Result, password field is displayed.
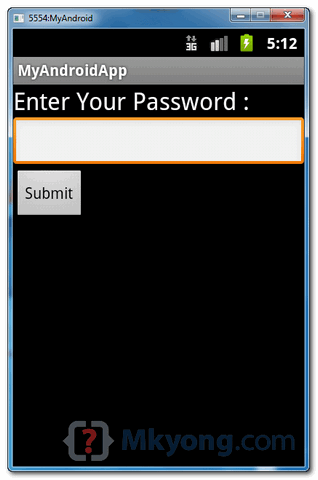
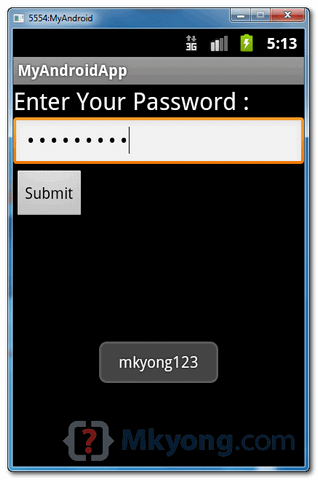
Download Source Code
Download it – Android-Password-Example.zip (15 KB)